I have been adding a lot of Qobuz releases to the MusizBrainz database the last week, but because of missing user-scripts (no API) it has been a little more time-consuming then importing from other sources like Discogs where there are userscripts.
(For more information about this read “Importing data from Discogs and others to MusicBrainz“)
So I had an idea, the FLAC-files from Qobuz comes very well tagged, so why not use these instead of copying the data from the web-page. especially copying the track-list from the website is a many-step manual process because it has to be corrected for the MusicBrainz praiser.
So I made a PowerShell script to make it easier.
The script
So, this is my script, copy it and save it as FlacMetaDatatoMusicBrainzHelper.ps1
on your disk.
But read on, because there is more needed.
<#
.Synopsis
Name: FlacMetaDatatoMusicBrainzHelper.ps1
A PowerShell script to display FLAC metadata in a copy paste friendly way for release-adding in MusicBrainz.
.DESCRIPTION
A script to make you more efficient when adding or editing releases on MusicBrainz with flac metadata as information source
For https://musicbrainz.org/release/add
The script will output suggestion Release information to Albuim, Artist, Release Group and Date based on metadata in first flac-file.
It will also output a tracklist matching what the MusicBrainz parser expects, just copy-paste into "Add Medium"
The script is relying on cmndlets in the AudioWorks.Commands module.
https://www.powershellgallery.com/packages/AudioWorks.Commands/1.2.0
Install-Module -Name AudioWorks.Commands
.NOTES
Original release Date: 28.12.2022
Author: Flemming Sørvollen Skaret
.LINK
https://github.com/flemmingss/
https://flemmingss.com
#>
#Requires -RunAsAdministrator
#Requires -Modules AudioWorks.Commands
Write-Host "* Flac MetaData to MusicBrainz Helper *" -ForegroundColor Magenta
Import-Module AudioWorks.Commands
do
{
[string]$Folder = Read-Host -Prompt "Path to release"
$Files = (Get-ChildItem -LiteralPath $Folder| Where-Object {$_.Extension -eq ".flac"}).Fullname
$PeriodCharacter = "."
$HyphenCharacter ="-"
$ParenthesisStartCharacter = "("
$ParenthesisEndCharacter = ")"
$Album = (Get-AudioFile -Path $Files[0] | Get-AudioMetadata).Album
$AlbumArtist = (Get-AudioFile -Path $Files[0] | Get-AudioMetadata).AlbumArtist
$Day = (Get-AudioFile -Path $Files[0] | Get-AudioMetadata).Day
$Month = (Get-AudioFile -Path $Files[0] | Get-AudioMetadata).Month
$Year = (Get-AudioFile -Path $Files[0] | Get-AudioMetadata).Year
Write-Host ""
Write-Host "### Release information ###" -ForegroundColor Yellow
Write-Host "Title.........: $Album"
Write-Host "Artist........: $AlbumArtist"
Write-Host "Release Group.: $Album"
Write-Host "Date..........: $Year$HyphenCharacter$Month$HyphenCharacter$Day"
Write-Host ""
Write-Host "### Tracklist ###" -ForegroundColor Yellow
foreach ($File in $Files)
{
$FileMetadata = Get-AudioFile -Path $File | Get-AudioMetadata
$AudioInfo = Get-AudioFile -Path $File | Get-AudioInfo
$FileTrackNumber = [int]$FileMetadata.TrackNumber
$FileArtist = $FileMetadata.Artist
$FileTitle = $FileMetadata.Title
$FilePlayLength = $AudioInfo.PlayLength
Write-Host $FileTrackNumber$PeriodCharacter $FileTitle $HyphenCharacter $FileArtist $ParenthesisStartCharacter$FilePlayLength$ParenthesisEndCharacter
}
}
until ($Folder -eq "exit")
The script is as described in the synopsis depending on a module called AudioWorks.Commands
. All commands in this article require administrator privileges in PowerShell.
How to run PowerShell as Administrator:
- Click the start-menu in Windows and search for “PowerShell”
- Right-click on “Windows PowerShell” and select “Run as administrator”
- Click “Yes” on “Do you want to allow this app to make changes to your device?”
- To verify that you run powershell as administrator, see the Windows title, it should be “Administrator: Windows PowerShell”
Run PowerShell as Administrator and run the following command: Install-Module -Name AudioWorks.Commands
.
You can then try to import the module by running Import-Module AudioWorks.Commands
in the same window.
Because the module repository is untrusted you will likely get an error “Import-Module : File C:\Program Files\WindowsPowerShell\Modules\AudioWorks.Commands\1.2.0\AudioWorks.Commands.psm1 cannot be loaded because running scripts is disabled on this system.
“. Run Set-ExecutionPolicy Bypass
to baypass this limitation.
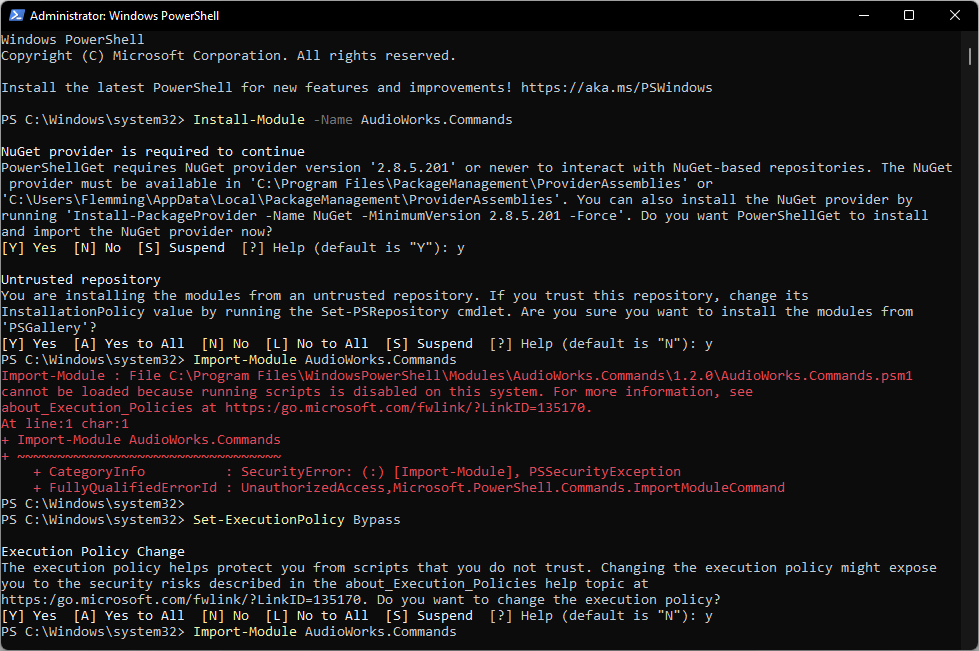
Next, just run the script and you will be asked for a path, input the album folder.
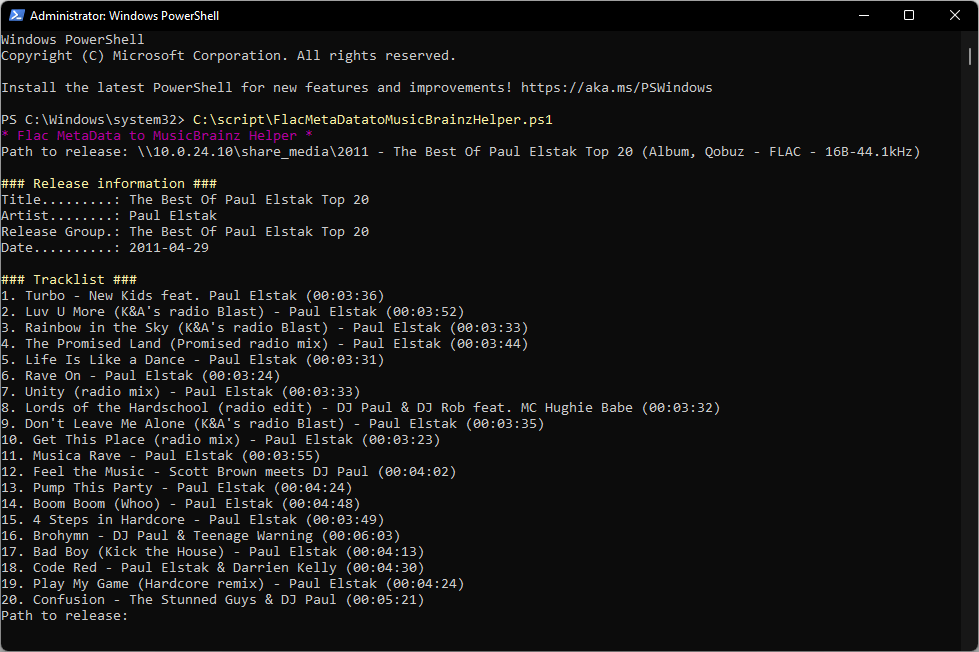
Here is your metadata 🙂
Use in practice:
I’ll let the pictures speak for themselves:
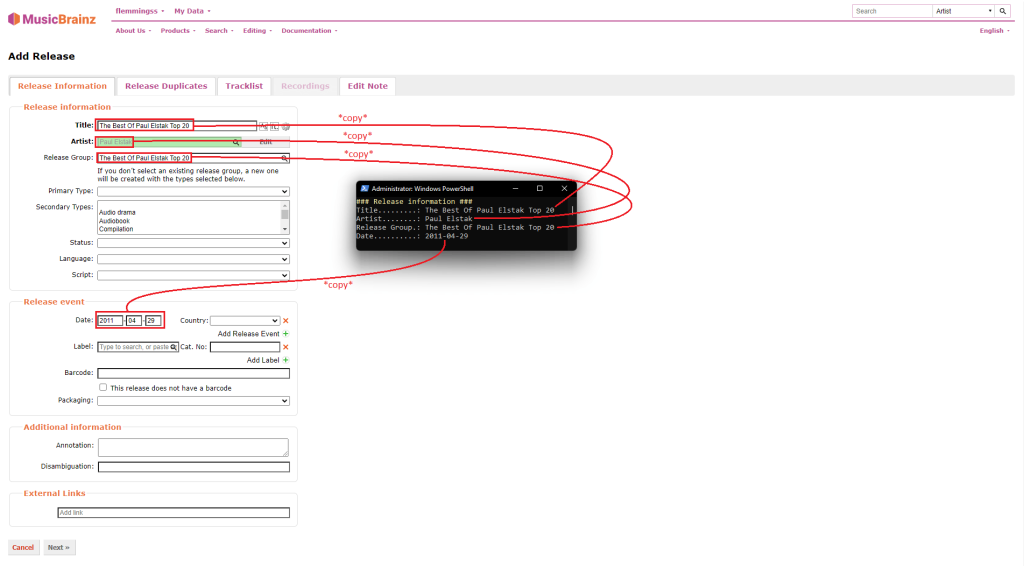
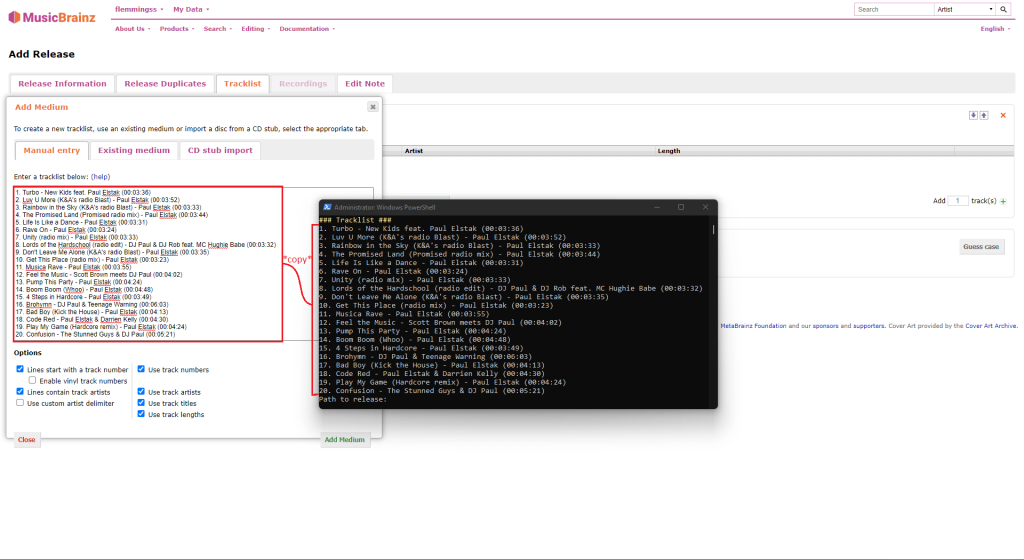
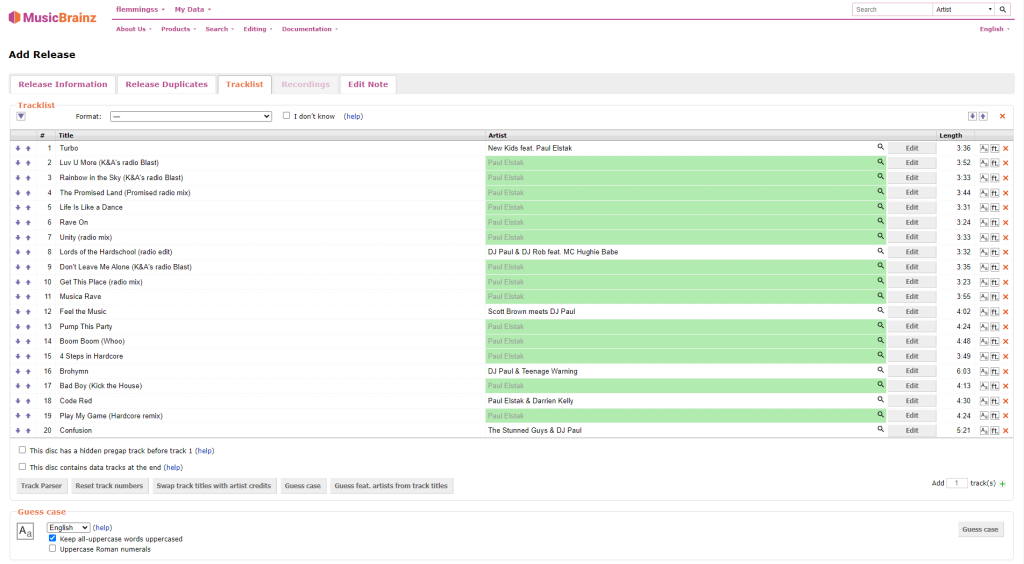
It is always a good idea to double-check the information after pasting.
Compatibility and bugs
have put no effort into error handling and compatibility in this script. Errors must therefore be expected. I am running PowerShell 5.1.22000.1335 and Windows 11 Pro.
Known bug/issuess:
- Can’t use square brackets [] in path/filename due to incompatibility in the AudioWorks module.
- Will output error if folder don’t contain any flac files
- Non-filled values will just be empty
- Release information is just based on first .flac file